Auto and Static Variable in C
What is Static Variable in C
In the C programming language, a static variable is a variable that retains its value across multiple function calls within the same scope. Unlike regular variables, which are typically allocated and deallocated each time a function is called, static variables are allocated once and persist throughout the program's execution.
Here's an example that demonstrates the usage of a static variable in C:
In this example, we have a function called increment() that contains a static variable named Chapter. The static variable is initialized to 0 when it is declared. Each time the increment() function is called, the chapter value is incremented by 1 and then printed using printf(). Since the variable is static, it retains its value between function calls.
When the program is executed, the increment() function is called three times from the primary () function. As a result, the output will be:
The Chapter variable persists in its value across the multiple invocations of the increment() function, allowing us to maintain and track the number of times the function has been called.
Characteristics of Static Variable
Static variables in programming languages like C and C++ have the following characteristics:
- Persistence of value: Static variables retain value across multiple function calls within the same scope. Once a static variable is assigned a value, it keeps that value until it is explicitly modified or the program terminates.
- Scope limitation:
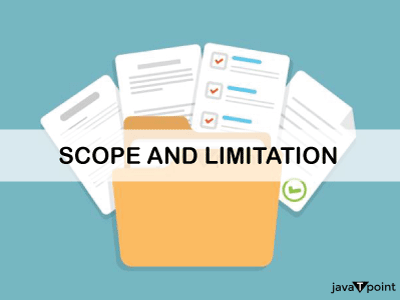
Static variables have block scope, meaning they are only accessible within the block or function in which they are declared. They cannot be accessed or modified outside that specific scope.
- Lifetime: Static variables have a lifetime that extends from the point of their declaration until the program terminates. They are allocated memory when the program starts and deallocated when it ends.
- Initialization: If a static variable is not explicitly initialized during declaration, it is automatically initialized to zero (or the equivalent null value) by default. However, if desired, you can assign an initial value to a static variable during declaration.
- Memory allocation:
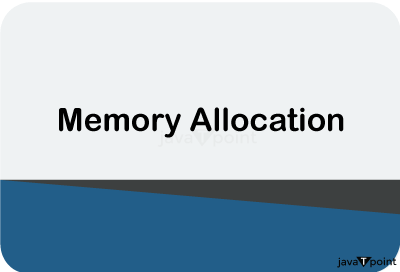
Static variables are allocated in a fixed location when the program starts, usually in a data segment. This memory location remains the same throughout the program's execution.
- Sharing of value: In multi-threaded programs, static variables are shared among all threads. This means that changes to a static variable made by one thread are visible to all other threads.
Advantages of Using Static Variables in Your C Code
Using static variables in your C code can provide several advantages, including:
- Persistent State: Static variables retain values across multiple function calls within the same scope. This allows you to maintain state information without the need for global variables. It can be beneficial when preserving data between function invocations or implementing counters or flags.
- Information Hiding: By declaring a static variable within a function or a file, you restrict its visibility to that specific scope. This promotes encapsulation and information hiding, preventing other parts of the code from directly accessing or modifying the variable. It helps maintain data integrity and reduces potential naming conflicts.
- Memory Efficiency: Static variables are allocated memory only once during program execution. Unlike automatic variables created and destroyed each time their enclosing block is entered and exited, static variables persist throughout the program's lifetime. This can save memory allocation and deallocation overhead, especially for large or frequently used data structures.
- Performance Optimization: In some cases, static variables can lead to performance optimizations. For example, caching frequently accessed data in a static variable can eliminate the need for redundant calculations or I/O operations.
Disadvantages of Using Static Variables in Your C Code
While static variables in C provide advantages, it's essential to be aware of their potential disadvantages as well. Here are some considerations:
- Reduced Flexibility: Static variables have limited scope and visibility, which can limit their flexibility. They are confined to the block or function in which they are declared, making it challenging to access or modify them from other parts of the code. This reduced flexibility can hinder code reuse and modularity in specific scenarios.
- Thread Safety Challenges:
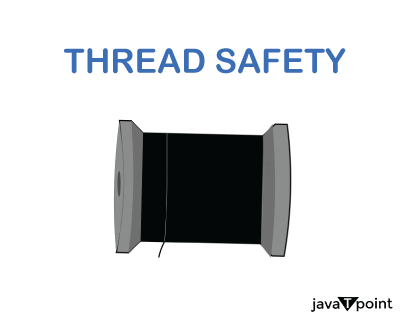
If multiple threads concurrently access or modify static variables without proper synchronization, it can lead to race conditions and unpredictable behavior. Careful consideration and synchronization mechanisms are necessary to ensure thread safety.
- Testing and Debugging Challenges: The encapsulation provided by static variables can make testing and debugging more challenging. This can hinder the ability to diagnose and fix issues effectively.
- Code Maintainability: Excessive use of static variables can make code harder to understand and maintain, especially in larger codebases. Overuse of static variables can lead to code that is difficult to modify and prone to errors.
- Global State Concerns: While static variables are not truly global, they can have similar implications if used carelessly. Excessive reliance on static variables can introduce a global state, making it more difficult to reason about the program's behavior. It can also hinder code reuse and make isolating and testing components independently harder.
How to Declare and Define a Static Variable in C
Declare the static variable in the desired scope (usually at the beginning of a block or function) using the static keyword. The declaration typically includes the variable type and an optional initialization.
Define the static variable by assigning it a value. The definition can be done at the same time as the declaration or separately.
Here's the general syntax for declaring and defining a static variable:
What is Auto Variable in C
In C, an auto variable is a local variable automatically created and destroyed within a block or function. The auto keyword is optional when declaring variables within a block or function, as it is the default storage class for local variables.
Example of Auto Variable in C
Output:
In this example, the printNumbers() function demonstrates the usage of auto variables. The num variable is declared as an auto variable and initialized with the value 10. It is then modified and printed. An inner block is also created within the function, and an inner auto variable innerNum is declared, initialized, modified, and printed. Once the inner block is exited, the innerNum variable is destroyed.
Auto variables are the default choice for local variables in C, and you typically don't need to use the auto keyword when declaring them explicitly. They provide automatic memory allocation and deallocation within the block or function scope, allowing you to work efficiently with temporary and local data.
How to Declare and Use Auto Variable in C
In C programming, declaring and using an auto variable is straightforward. However, it's important to note that the auto keyword is optional since local variables are auto variables by default. Here's how you can declare and use an auto variable:
Output
In this example, the printNumber() function declares an auto variable named num and initializes it with 10. The value of the auto variable is then printed using printf(). Since local variables are auto variables by default, explicitly using the auto keyword in the declaration is unnecessary.
Difference Between Auto Variable and Static Variable
The critical differences between auto variables and static variables in C are as follows:
- Storage Duration: Auto variables have automatic storage duration, which means they are created and destroyed automatically when the block or function in which they are defined is entered and exited, respectively. On the other hand, static variables have static storage duration, which means they are created and initialized once and retain their value across multiple function calls.
- Memory Allocation: Auto variables are allocated on the stack, a temporary storage area that grows and shrinks as blocks or functions are entered and exited. On the other hand, static variables are allocated in the data segment of memory and persist throughout the program's execution.
- Initialization: Auto variables can be uninitialized or initialized with a value at the time of declaration. If left uninitialized, auto variables contain garbage values until assigned a specific value. Static variables are initialized to zero by default if no explicit initialization is provided.
- Scope: Auto variables have block scope, which means they are visible and accessible only within the block or function in which they are declared. They cannot be accessed from outside the block or function. They can be accessed by other functions within the same file using the extern keyword.
- Persistence of Value: Auto variables do not retain value across multiple function calls. Static variables, however, retain their value between function calls. They maintain their value across multiple invocations of the function, allowing them to preserve state or data across different function calls.
|